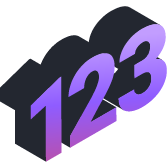
Numbers only regex (digits only) C#
Numbers only (or digits only) regular expressions can be used to validate if a string contains only numbers.
Basic numbers only regex
Below is a simple regular expression that allows validating if a given string contains only numbers:
Test it!
/^\d+$/
True
False
Enter a text in the input above to see the result
Example code in C#:
Real number regex
Real number regex can be used to validate or exact real numbers from a string.
Test it!
/^(?:-(?:[1-9](?:\d{0,2}(?:,\d{3})+|\d*))|(?:0|(?:[1-9](?:\d{0,2}(?:,\d{3})+|\d*))))(?:.\d+|)$/
True
False
Enter a text in the input above to see the result
Example code in C#:
Test it!
True
False
Enter a text in the input above to see the result
Notes on number only regex validation
In C# you can also validate number by trying to parse it: