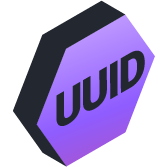
UUID regex C#
UUID is a 128-bit label used for identifications in computer systems.
UUID regex
Below is a simple UUID regular expression that will work most of the time:
Test it!
/^[0-9a-f]{8}-[0-9a-f]{4}-[0-5][0-9a-f]{3}-[089ab][0-9a-f]{3}-[0-9a-f]{12}$/i
True
False
Enter a text in the input above to see the result
Example code in C#:
Test it!
True
False
Enter a text in the input above to see the result
Test it!
True
False
Enter a text in the input above to see the result