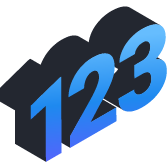
Numbers only (digits only)
Numbers only (or digits only) regular expressions can be used to validate if a string contains only numbers.
Basic numbers only regex
Below is a simple regular expression that allows validating if a given string contains only numbers:
Test it!
This is some text inside of a div block.
True
False
Enter a text in the input above to see the result
Example code in Python:
Real number regex
Real number regex can be used to validate or exact real numbers from a string.
Test it!
This is some text inside of a div block.
True
False
Enter a text in the input above to see the result
Example code in Python:
Test it!
This is some text inside of a div block.
True
False
Enter a text in the input above to see the result
Notes on number only regex validation
In Python you can also validate number by using isnumeric method: